06/01/2024
Blog Detail
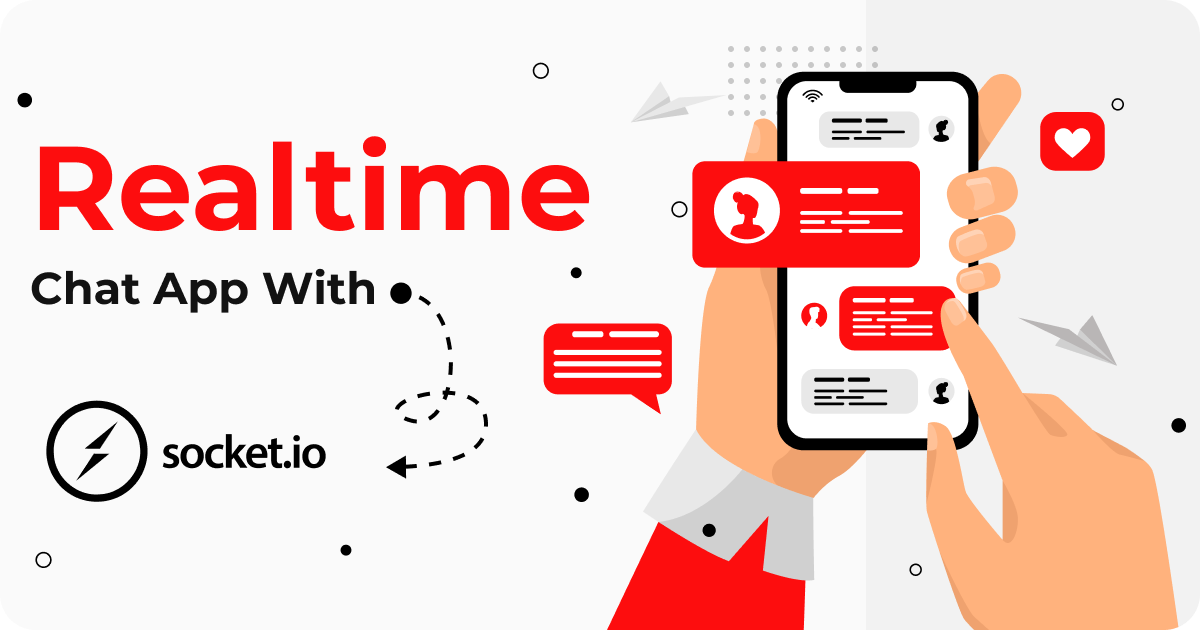
Real-time data transfer Using Socket.io
5:05 AM
Introduction
Whether you are building a chat application, a video sharing app, or even a video conferencing app, Socket.io provides a connection between your clients, allowing for lightning speed data transfer between them.
It consists of both the client side and the server side, with the server side handling different connections and information dispersion. You should know that Socket.io is not a WebSocket, but rather a custom real-time transfer protocol implementation built on other real-time protocols.
This article covers the basics of Socket.io, from setting up a connection to connecting your client side to the server side.
What is WebSocket?
WebSocket is a web communication protocol that allows for the transfer of data from the client side to the server side (and from the server side to the client side) once a channel between them is created. This connection persists until the channel is terminated. Unlike the HTTPS protocol, WebSocket allows for data transfer in both directions.
WebSockets can be used to build out anything from a real-time trading application to a chat application, or just about anything that requires efficient, real-time data transfer.
What are sockets and Socket.io?
Sockets are connections achieved between given points in a network. A connection in a socket persists once created until it is terminated. Socket connections can be a server to the client, client to server, or between two clients or servers.
Socket.io is a JavaScript library that works similarly to WebSockets. Data transfer is done via an open connection allowing for real-time data exchange. Each connection, also known as a socket, consists of two parts: The server side and the client side. Socket.io makes use of Engine.io to achieve server-side connection with Engine.io-client for the client side.
Data transfer in Socket.io is mostly done in the form of a JSON (JavaScript Object Notation), so we will be using the JSON format for this article.
Installation and setup
Because Socket.io needs both a server side and a client side to achieve a connection, we will be making installations for the both (server and client) starting with the server side and then moving to the client side.
Server side
Ensure that you have Node.js and node package manager (npm) already installed in your system. If not, proceed to the Node.js website to learn more about installation.
In your command line, run the following command:
npm install express Socket.io
This will install the necessary dependencies.
The next step is to set up our server. Proceed to require Socket.io, Express, and the HTTP module as shown below in your index.js file. We will be using the HTTP module to create our server instead of Express in order to pass our server to Socket.io easily:
const express = require("express"); const http = require("http"); const socketio = require("socket.io"); const app = express(); const PORT = process.env.PORT || 5000; const server = http.createServer(app); io = socketio(server); server.listen(PORT, () => { console.log(`server running at port ${PORT}`); });
As shown above, we successfully created our server. We can start our server by running the following:
node index.js
Our server should be up and running at port 5000. Notice that our server was passed to Socket.io.
Client side
For the client-side setup, let’s import the Socket.io script into our index.html file:
Proceed to connect your client side to the server side as shown below:
let ENDPOINT = "http://localhost:5000" let socket = io.connect(ENDPOINT);
Alternatively, you can install socket.io-client
for your client side if you’re making use of React:
npm install socket.io-client
You can then proceed to import and create a connection like so:
import React from "react"; import io from "socket.io-client"; let ENDPOINT = "http://localhost:5000" let socket = io(ENDPOINT);
Socket connection and disconnection
We have succeeded in setting up both our server side and client side. Now, once the client side is connected, we are notified of this connection on the server side. Socket.io helps us handle this connection as such:
io.on("connection", (socket) => { // your code snippet })
This event, equipped with a callback function, gets fired when a connection occurs.
For more complex applications, you might want to create specific rooms for different groups of users. This feature can be easily achieved using Socket.io. You can join particular rooms using the join method once a connection is achieved:
io.on("connection", (socket) => { let roomID = 2436 socket.join(roomID) })
The disconnect event is fired once a disconnection occurs:
io.on("connection", (socket) => { socket.on("disconnect", () => { }); })
Event emission and broadcasting
Socket.io allows users to emit events and listen for them on the other end. Event emission can happen on both the server side and the client side. A “join” event, for instance, can be emitted on the client side during a connection:
socket.emit("join", { name: “peter paul”}, (err) => { if (err) { alert(err); } });
Notice that the event is emitted along with a payload in the form of a JSON, which contains the name of the user that is currently joining the connection.
Proceed to handle this event on the server side:
socket.on("join", ({ name }, callback) => { if (error) { callback(error); } else { let roomID = 2436 socket.join(roomID) console.log(name) });
You can also choose to broadcast information to everyone in a specific room during event handling:
socket.on("join", ({ name }, callback) => { if (error) { callback(error); } else { let roomID = 2436 socket.join(roomID) socket.broadcast.to(roomID).emit("adminMessage", { name: "admin", content: `${name} has joined`, }); } });
All our server-side code should look like this at this point:
const express = require("express"); const http = require("http"); const socketio = require("socket.io"); const app = express(); const PORT = process.env.PORT || 5000; const server = http.createServer(app); io = socketio(server); io.on("connection", (socket) => { socket.on("join", ({ name }, callback) => { if (error) { callback(error); } else { let roomID = 2436 socket.join(roomID) console.log(name) socket.broadcast.to(roomID).emit("adminMessage", { name: "admin", content: `${name} has joined`, }); }); socket.on("disconnect", () => { }); }) server.listen(PORT, () => { console.log(`server running at port ${PORT}`); });
The client side should be structured as such:
let ENDPOINT = "http://localhost:5000" let socket = io.connect(ENDPOINT); socket.emit("join", { name: “peter paul”}, (err) => { if (err) { alert(err); } });
Recent Posts
- Harnessing the Power of Swagger in Laravel: A Comprehensive Guide
- Mastering API Testing Unveiling the Power of Postman and Beyond
- Unleashing Creativity Exploring the Best Design Tools in 2024
- Transformative Learning: Building an AI-Enhanced E-Learning Platform with Python
- Choosing the Right Server-Side Scripting Language PHP vs Node.js
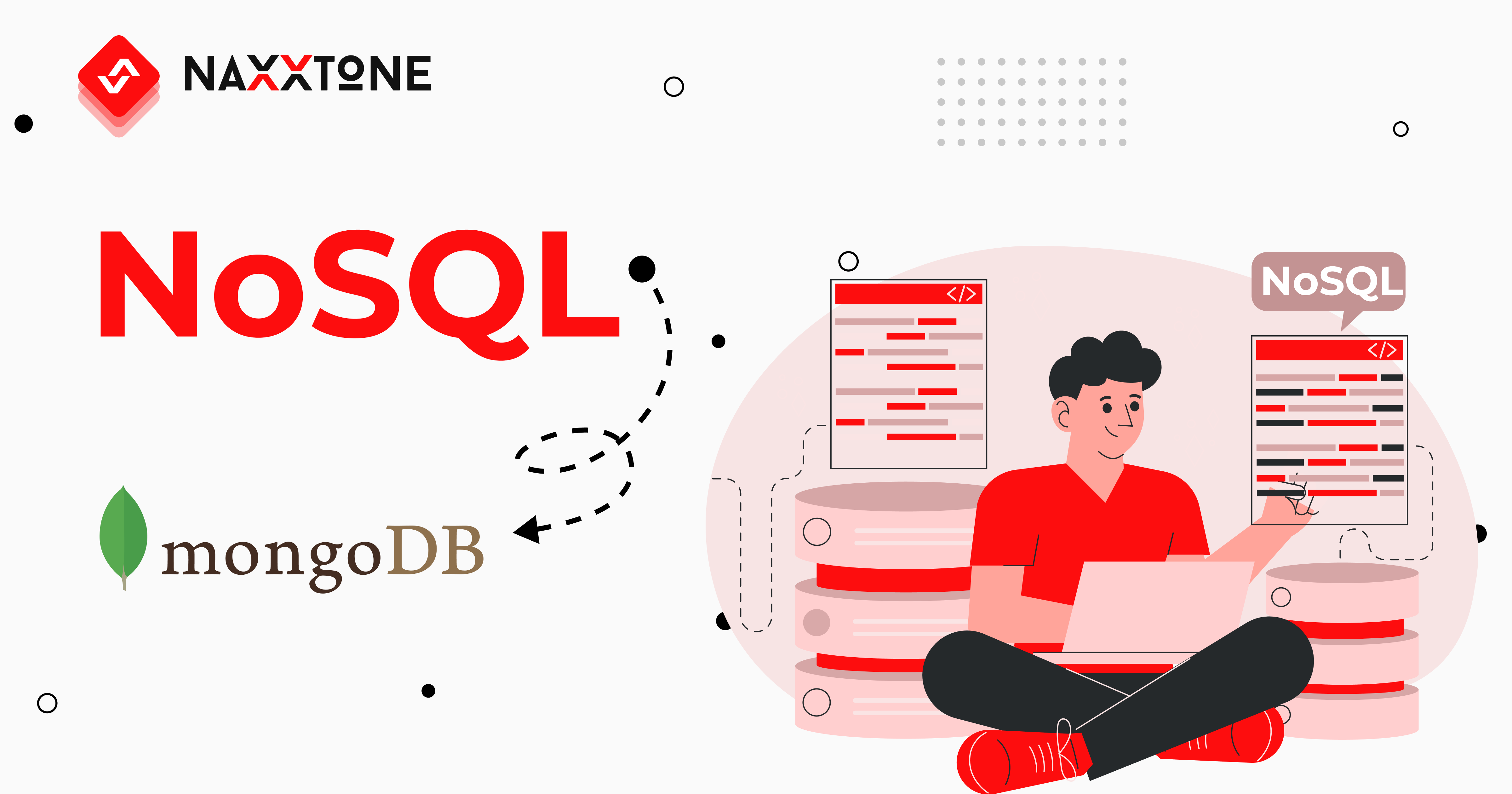
Embracing the Power of NoSQL Databases , Revolutionizing Data Management
NoSQL databases have undoubtedly become a game-changer in the realm of data management, offering a dynamic and scalable alternative to traditional relational databases. As technology continues to advance and data volumes grow, the versatility of NoSQL databases positions them as a pivotal tool for developers and businesses looking to unlock new possibilities in data storage and retrieval. By embracing the power of NoSQL, we can build more resilient, scalable, and performance-oriented applications that meet the demands of the modern digital landscape.
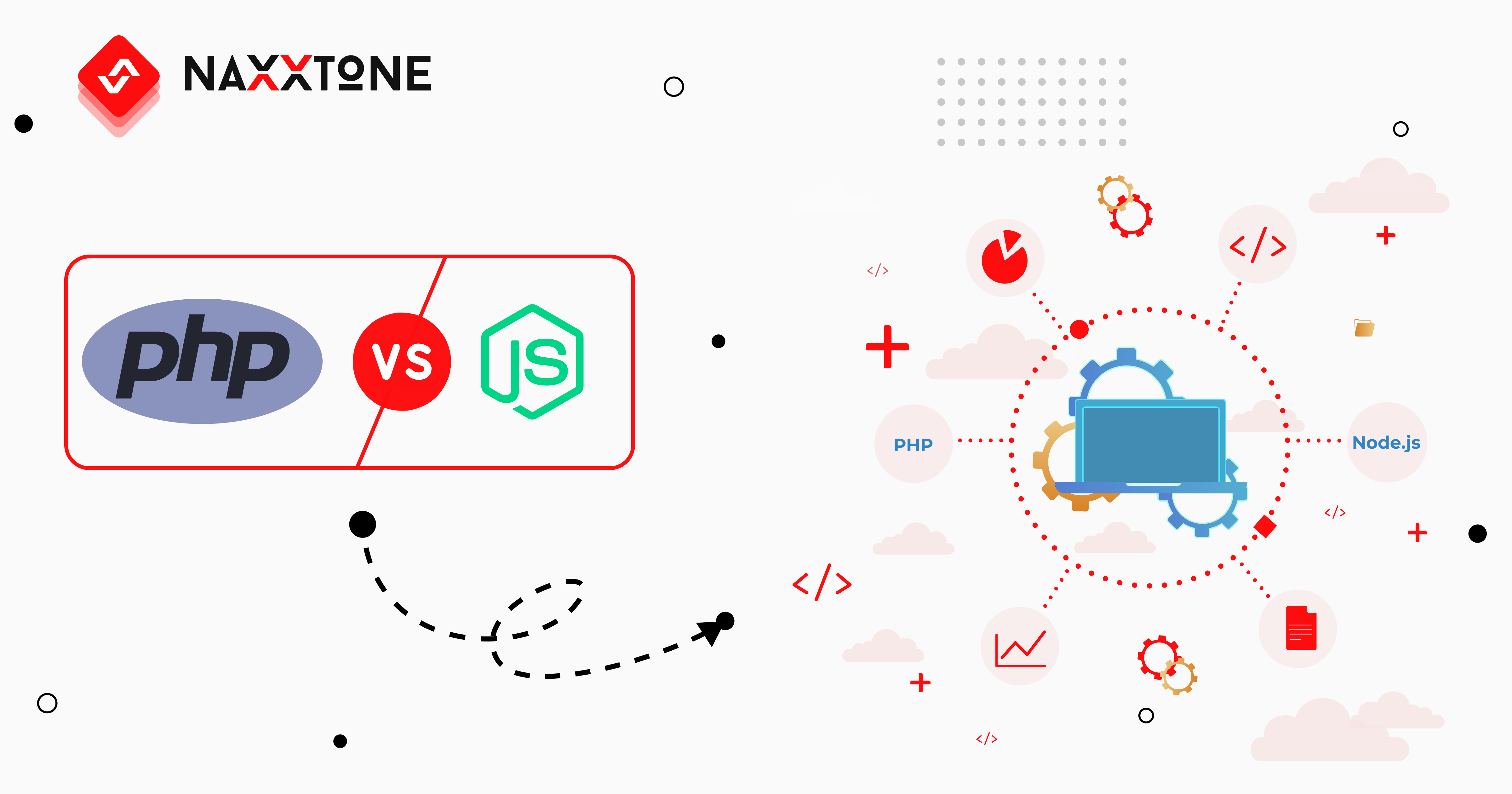
Choosing the Right Server-Side Scripting Language PHP vs Node.js
Choosing between PHP and Node.js ultimately depends on the nature of your project, your team's expertise, and the specific requirements you need to address. While PHP continues to be a robust choice for traditional web development, Node.js has emerged as a powerful contender for modern, real-time applications. By carefully evaluating the strengths and use cases of each, you can make an informed decision that aligns with your development goals and project needs.
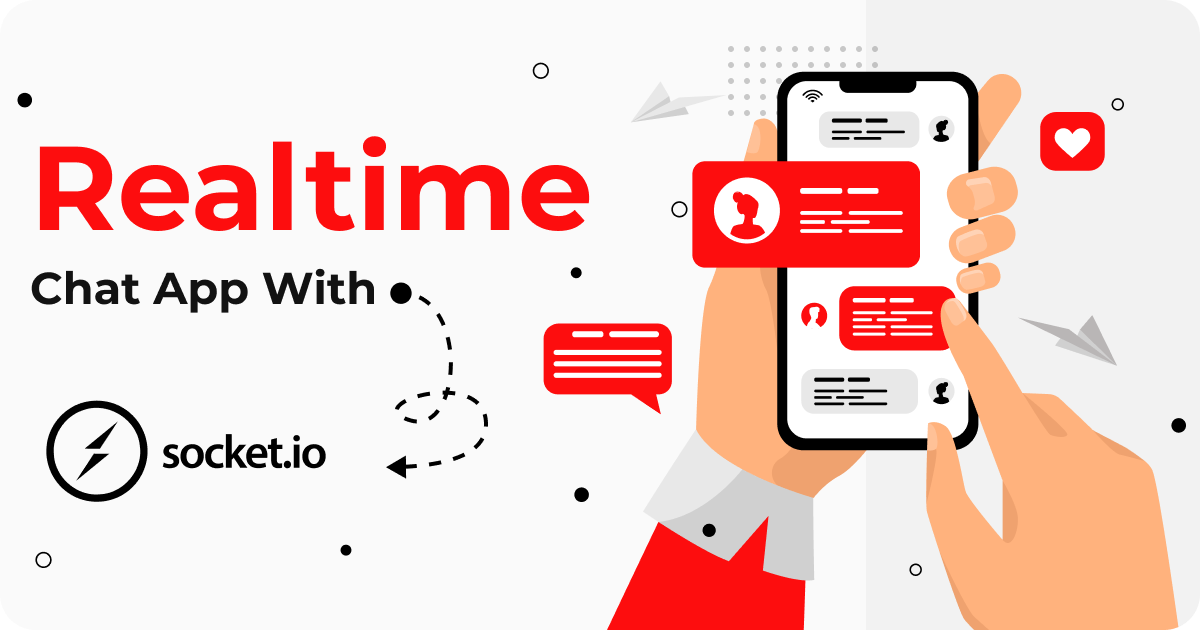
Real-time data transfer Using Socket.io
Socket.io, with all its simplicity, has become a top choice for developers in building applications that require real-time data transfer. With almost all modern browsers supporting WebSocket, Socket.io is poised to get even bigger
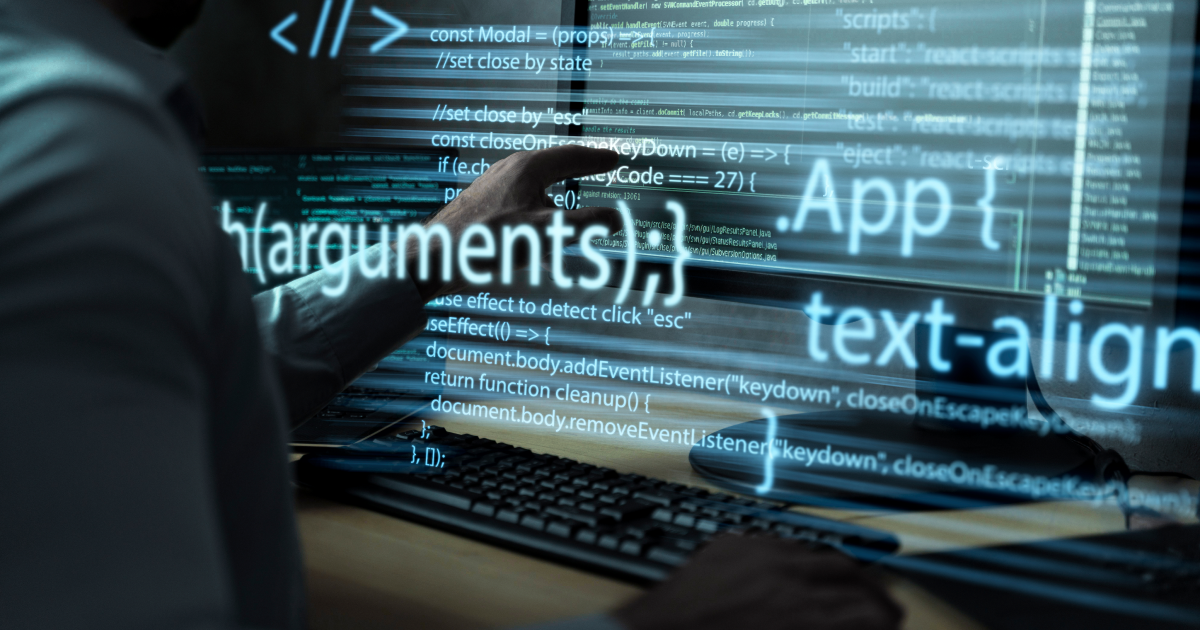
Spelunking in Node Modules
Spelunking in node modules refers to delving into the node modules directory of a Node.js project to understand or debug the installed nested dependencies.
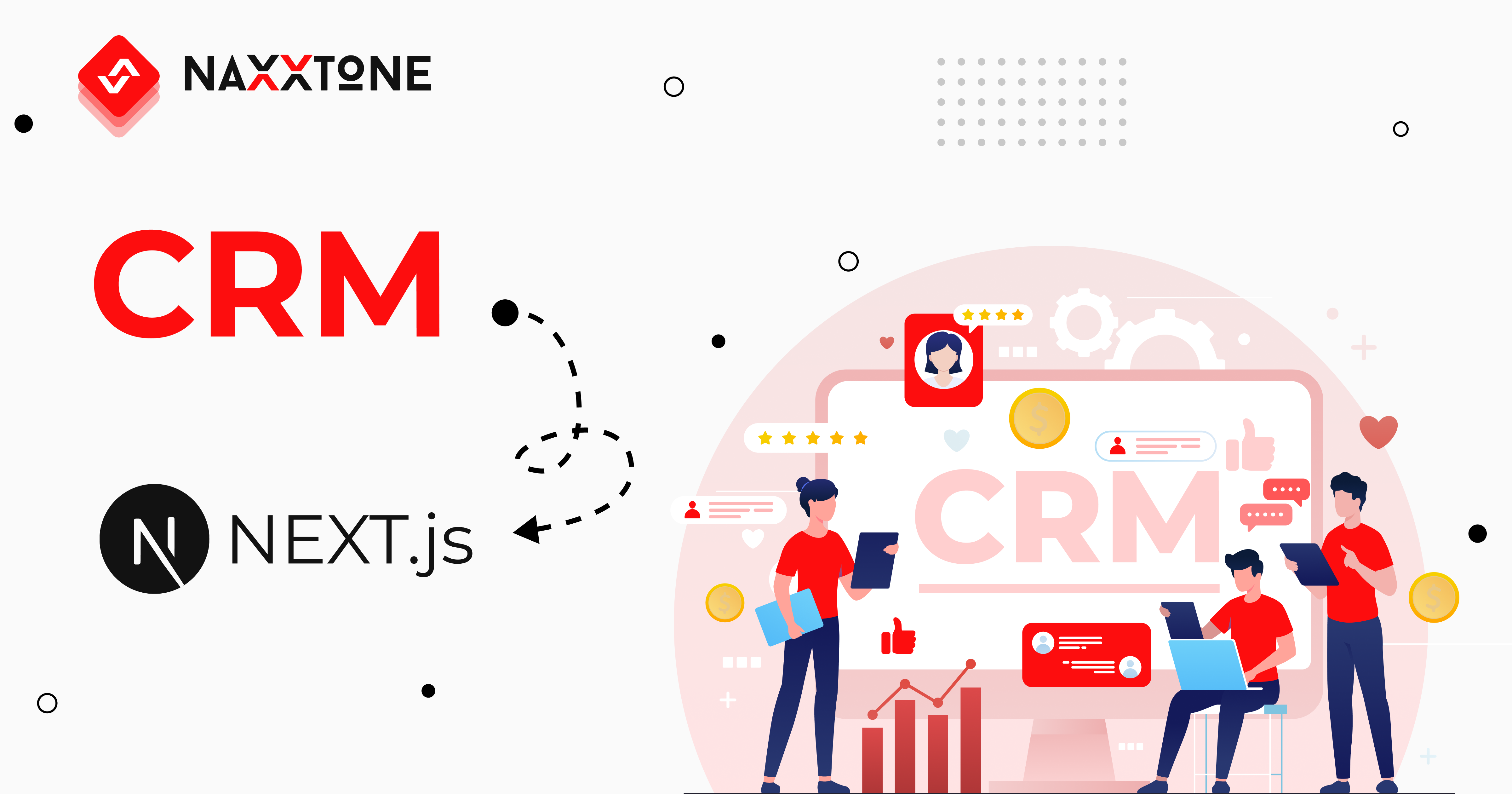
Unleashing the Power of CRM Development with Next.js: A Comprehensive Guide
As businesses continue to evolve, the need for efficient CRM systems becomes more pronounced. Next.js provides a solid foundation for developing dynamic, high-performance CRM applications that can propel your organization to new heights. By following the comprehensive guide outlined in this blog, you'll be well-equipped to embark on your Next.js CRM development journey and create a customer-centric solution that sets your business apart.